uselessmail
Junior Member level 3
Hi there!
I have made a simple program to generate an MLS signal (Maximum length Sequence). Its a type of a PRBS signal with some additional properties. In the code, I found it easier to simply write three different architectures in the same entity. I was wondering if I could probably write the entire code within a single architecture. Is there any benefit of doing so? Or is easier to just keep the code as it is?
Also, I need to get the digital output serially through a single port, for eg through the SMA connector on my board. But I cant do that since the output is stored in a signal array of 0 to 9 and I'm only able to connect it to 10 different output ports!
In other words, I need an output from a single SMA connector which will look something like this...
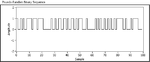
Hope someone can help me figure this out!
I have made a simple program to generate an MLS signal (Maximum length Sequence). Its a type of a PRBS signal with some additional properties. In the code, I found it easier to simply write three different architectures in the same entity. I was wondering if I could probably write the entire code within a single architecture. Is there any benefit of doing so? Or is easier to just keep the code as it is?
Also, I need to get the digital output serially through a single port, for eg through the SMA connector on my board. But I cant do that since the output is stored in a signal array of 0 to 9 and I'm only able to connect it to 10 different output ports!
In other words, I need an output from a single SMA connector which will look something like this...
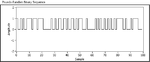
Hope someone can help me figure this out!
Code:
entity mlbs is
Port ( clock : in STD_LOGIC;
reset : in STD_LOGIC;
data_out : inout STD_LOGIC_VECTOR (9 downto 0) );
end mlbs;
architecture Behavioral of mlbs is
component mlbs_clk
port
(-- Clock in ports
CLK_IN1 : in std_logic;
-- Clock out ports
CLK_OUT1 : out std_logic
);
end component;
signal clk_out : std_logic;
signal lfsr_reg : std_logic_vector (9 downto 0);
begin
clkmodle1 : mlbs_clk
port map
(-- Clock in ports
CLK_IN1 => clock,
-- Clock out ports
CLK_OUT1 => clk_out);
process(clk_out)
variable lfsr_tap: std_logic;
begin
if rising_edge(clk_out) then
if reset = '1' then
lfsr_reg <= (others => '1');
else
lfsr_tap := lfsr_reg(6) xor lfsr_reg(9);
lfsr_reg <= lfsr_reg(8 downto 0) & lfsr_tap;
end if;
end if;
end process;
data_out <= lfsr_reg;
end Behavioral;
architecture Behavioral_2 of mlbs is
component mlbs_clk
port
(-- Clock in ports
CLK_IN1 : in std_logic;
-- Clock out ports
CLK_OUT1 : out std_logic
);
end component;
signal clk_out : std_logic;
signal lfsr_reg : std_logic_vector (9 downto 0);
begin
clkmodle2 : mlbs_clk
port map
(-- Clock in ports
CLK_IN1 => clock,
-- Clock out ports
CLK_OUT1 => clk_out);
process(clk_out)
variable lfsr_tap: std_logic;
begin
if rising_edge(clk_out) then
if reset = '1' then
lfsr_reg <= (others => '1');
else
lfsr_tap := lfsr_reg(3) xor lfsr_reg(5);
lfsr_reg <= lfsr_reg(8 downto 0) & lfsr_tap;
end if;
end if;
end process;
data_out <= lfsr_reg;
end Behavioral_2;
architecture Behavioral_3 of mlbs is
component mlbs_clk
port
(-- Clock in ports
CLK_IN1 : in std_logic;
-- Clock out ports
CLK_OUT1 : out std_logic
);
end component;
signal clk_out : std_logic;
signal lfsr_reg : std_logic_vector (9 downto 0);
begin
clkmodle3 : mlbs_clk
port map
(-- Clock in ports
CLK_IN1 => clock,
-- Clock out ports
CLK_OUT1 => clk_out);
process(clk_out)
variable lfsr_tap: std_logic;
begin
if rising_edge(clk_out) then
if reset = '1' then
lfsr_reg <= (others => '1');
else
lfsr_tap := lfsr_reg(1) xor lfsr_reg(6);
lfsr_reg <= lfsr_reg(8 downto 0) & lfsr_tap;
end if;
end if;
end process;
data_out <= lfsr_reg;
end Behavioral_3;