Alper özel
Member level 1
- Joined
- Feb 28, 2015
- Messages
- 40
- Helped
- 0
- Reputation
- 0
- Reaction score
- 0
- Trophy points
- 6
- Location
- Turkey / Scotland
- Activity points
- 487
I am trying to write an SPI master module on my own to learn FPGA-Verilog efficiently.
This is my spi_master module:
and this is my test module:
The problem is test module seem like not communication with the spi module(as seen in the picture). When you check the ISim diagrams, clk is working and any initialization on test module is working(Start, miso) but Tx_data is not passed to mosi and sck is not dividing clk. Where am I doing wrong? I can understand the other s not working but sck is pretty easy don't you think?
I appreciate any help. Thanks.
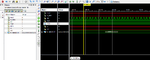
This is my spi_master module:
Code:
module spi_master(
input [15:0] tx_data,
output [15:0] rx_data,
output mosi,
input miso,
output cs,
output sck,
input start,
input clk
);
wire [15:0] tx_data;
reg [3:0] tx_counter;
reg [3:0] rx_counter;
wire start;
reg cs;
reg mosi;
reg [15:0] rx_data;
wire miso;
reg sck;
reg [3:0] clk_divider;
initial begin
clk_divider [3:0] = 4'b0;
tx_counter [3:0] = 4'b0;
rx_counter [3:0] = 4'b0;
cs = 1;
sck = 0;
end
always @(start) begin
if (clk_divider == 4'b1000) begin // divided by 8
sck = ~sck;
end
if (clk_divider >= 4'b1000) begin
clk_divider [3:0] = 4'b0;
end
else begin
#1 clk_divider = clk_divider + 4'b1;
end
end
always @(negedge sck) begin
if (cs == 0 && tx_counter != 4'b1111) begin
#(clk_divider/2) mosi <= tx_data[tx_counter];
#(clk_divider/2 + 1) tx_counter <= tx_counter + 4'b1;
end
else if(cs == 0 && tx_counter == 4'b1111) begin
#(clk_divider/2) cs <= 1'b1;
end
else if (cs == 1 && tx_counter == 0) begin
#(clk_divider/2) cs <= 1'b0;
end
end
always @(posedge sck) begin
if (cs == 0 && tx_counter <= 4'b1111 && tx_counter >= 4'b0001) begin
#(clk_divider/2) rx_data[rx_counter] <= miso;
#(clk_divider/2 + 1) rx_counter <= rx_counter + 4'b1;
end
end
endmodule
and this is my test module:
Code:
`include "spi_master.v"
module spi_master_tb(
);
reg clk;
reg start;
wire mosi;
reg miso;
reg cs;
reg sck;
reg [15:0] tx_data;
//reg [15:0] rx_data;
initial begin
clk = 0;
tx_data = 16'hF0AA;
#20 start = 1'b1;
miso = 1;
#1000 $finish;
end
always begin
#1 clk = ~clk;
end
spi_master SPI_block(
tx_data,
rx_data,
mosi,
miso,
cs,
sck,
start,
clk
);
endmodule
The problem is test module seem like not communication with the spi module(as seen in the picture). When you check the ISim diagrams, clk is working and any initialization on test module is working(Start, miso) but Tx_data is not passed to mosi and sck is not dividing clk. Where am I doing wrong? I can understand the other s not working but sck is pretty easy don't you think?
I appreciate any help. Thanks.
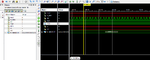