lylemalone
Newbie level 1

- Joined
- Apr 9, 2014
- Messages
- 1
- Helped
- 0
- Reputation
- 0
- Reaction score
- 0
- Trophy points
- 1
- Activity points
- 13
Hi all, I'm a beginner when it comes to verilog and I'm hoping to get some feedback on a project I'm working on:
Description: The circuit block diagram that can display four seven-segment displays with a shift register and a display buffer is shown in Figure P2.1. When the enable signal is low, the data to be displayed can be shifted in from the Din pin. When the enable signal goes high, the data cannot be shifted in. On the rising edge of the enable signal, the display data is transferred to the display data register and update the four seven-segment displays. The most significant bit is shifted in first.
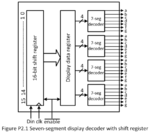
Below is the code I've come up with. I hope I made a solid approach at this but I'm not sure and can't test it at the moment. The way i split the sixteen bits on the shift register into each of the four decoders seemed simple enough, but i feel like perhaps I oversimplified the problem?
Description: The circuit block diagram that can display four seven-segment displays with a shift register and a display buffer is shown in Figure P2.1. When the enable signal is low, the data to be displayed can be shifted in from the Din pin. When the enable signal goes high, the data cannot be shifted in. On the rising edge of the enable signal, the display data is transferred to the display data register and update the four seven-segment displays. The most significant bit is shifted in first.
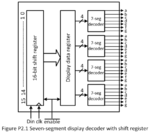
Below is the code I've come up with. I hope I made a solid approach at this but I'm not sure and can't test it at the moment. The way i split the sixteen bits on the shift register into each of the four decoders seemed simple enough, but i feel like perhaps I oversimplified the problem?
Code:
module bcd_to_seven_seg(bcd1,bcd2,bcd3,bcd4,din,clk,enable, qout);
output reg [3:0] bcd1, bcd2, bcd3, bcd4; //declaring each 4-bit decoder
input din, clk, enable;
output reg [15:0] qout; //shift register values
always @(posedge clk && !enable) begin //start shifting register on
qout[0] = qout[1]; //on positive clock edge and
qout[1] = qout[2]; // when enable is low
qout[2] = qout[3];
qout[3] = qout[4];
qout[4] = qout[5];
qout[5] = qout[6];
qout[6] = qout[7];
qout[7] = qout[8];
qout[8] = qout[9];
qout[9] = qout[10];
qout[10] = qout[11];
qout[11] = qout[12];
qout[12] = qout[13];
qout[13] = qout[14];
qout[14] = qout[15];
qout[15] = din;
end
always @(posedge enable) begin //transfer data to decoders on
bcd1[3] = qout[15]; //enable's positive edge
bcd2[3] = qout[11];
bcd3[3] = qout[7];
bcd4[3] = qout[3];
bcd1[2] = qout[14];
bcd2[2] = qout[10];
bcd3[2] = qout[6];
bcd4[2] = qout[2];
bcd1[1] = qout[13];
bcd2[1] = qout[9];
bcd3[1] = qout[5];
bcd4[1] = qout[1];
bcd1[0] = qout[12];
bcd2[0] = qout[8];
bcd3[0] = qout[4];
bcd4[0] = qout[0];
end
always @(bcd1 | bcd2 | bcd3 | bcd4) begin
case (bcd1)
0: bcd1 = 7'b0111111;
1: bcd1 = 7'b0000110;
2: bcd1 = 7'b1011011;
3: bcd1 = 7'b1001111;
4: bcd1 = 7'b1100110;
5: bcd1 = 7'b1101101;
6: bcd1 = 7'b1111101;
7: bcd1 = 7'b0000111;
8: bcd1 = 7'b1111111;
9: bcd1 = 7'b1101111;
default: bcd1 = 7'b0000000;
endcase
case (bcd2)
0: bcd2 = 7'b0111111;
1: bcd2 = 7'b0000110;
2: bcd2 = 7'b1011011;
3: bcd2 = 7'b1001111;
4: bcd2 = 7'b1100110;
5: bcd2 = 7'b1101101;
6: bcd2 = 7'b1111101;
7: bcd2 = 7'b0000111;
8: bcd2 = 7'b1111111;
9: bcd2 = 7'b1101111;
default: bcd2 = 7'b0000000;
endcase
case (bcd3)
0: bcd3 = 7'b0111111;
1: bcd3 = 7'b0000110;
2: bcd3 = 7'b1011011;
3: bcd3 = 7'b1001111;
4: bcd3 = 7'b1100110;
5: bcd3 = 7'b1101101;
6: bcd3 = 7'b1111101;
7: bcd3 = 7'b0000111;
8: bcd3 = 7'b1111111;
9: bcd3 = 7'b1101111;
default: bcd3 = 7'b0000000;
endcase
case (bcd4)
0: bcd4 = 7'b0111111;
1: bcd4 = 7'b0000110;
2: bcd4 = 7'b1011011;
3: bcd4 = 7'b1001111;
4: bcd4 = 7'b1100110;
5: bcd4 = 7'b1101101;
6: bcd4 = 7'b1111101;
7: bcd4 = 7'b0000111;
8: bcd4 = 7'b1111111;
9: bcd4 = 7'b1101111;
default: bcd4 = 7'b0000000;
endcase
end
endmodule