NIKO BELLIC
Newbie level 6
- Joined
- Aug 1, 2011
- Messages
- 14
- Helped
- 6
- Reputation
- 12
- Reaction score
- 5
- Trophy points
- 1,283
- Location
- Kansas City, MO
- Activity points
- 1,389
Hi all,
I want to operate a stepper motor in different modes using single keypad. That is, based on Full step, Half step, high torque, low torque and its direction. For this, in proteus ISIS, I've created a keypad consisting of all those operations. With my basic knowledge, I've prepared a code for it. But, that ain't working properly. The main problem is, once I press a key in the keypad, it starts doing that corresponding operation but if I press another key it is just ignoring... I could not able to program it properly... Can someone help me..!!
Microcontroller - ATmega 16
Compiler - WinAVR 20100110
Circuit Diagram:
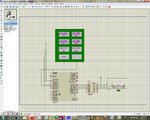
I want to operate a stepper motor in different modes using single keypad. That is, based on Full step, Half step, high torque, low torque and its direction. For this, in proteus ISIS, I've created a keypad consisting of all those operations. With my basic knowledge, I've prepared a code for it. But, that ain't working properly. The main problem is, once I press a key in the keypad, it starts doing that corresponding operation but if I press another key it is just ignoring... I could not able to program it properly... Can someone help me..!!
Microcontroller - ATmega 16
Compiler - WinAVR 20100110
Code:
// This program is used to control the stepper motor in desired direction and torque as given by inputs
#include <avr/io.h>
#include <util/delay.h>
#define keypad PORTA
#define stepper PORTD
void main()
{
DDRA=0x0F; // configuring keypad pins
DDRD=0xFF; // configuring ULN2003A pins as output
keypad=0xF0;
scan_keypad();
}
scan_keypad()
{
unsigned char value;
while(1)
{
keypad=0xF0;
value=PINA;
if(value!=0xF0)
{
check_row_1();
check_row_2();
check_row_3();
check_row_4();
}
}
}
check_row_1()
{
keypad=0b11111110;
_delay_ms(4);
if(bit_is_clear(PINA,PA4))
full_step_high_torque_left();
if(bit_is_clear(PINA,PA5))
full_step_high_torque_right();
}
check_row_2()
{
keypad=0b11111101;
_delay_ms(4);
if(bit_is_clear(PINA,PA4))
full_step_low_torque_left();
if(bit_is_clear(PINA,PA5))
full_step_low_torque_right();
}
check_row_3()
{
keypad=0b11111011;
_delay_ms(4);
if(bit_is_clear(PINA,PA4))
half_step_left();
if(bit_is_clear(PINA,PA5))
half_step_right();
}
check_row_4()
{
keypad=0b11110111;
_delay_ms(4);
if(bit_is_clear(PINA,PA4))
stop();
if(bit_is_clear(PINA,PA5))
;
}
full_step_high_torque_left()
{
while(1)
{
stepper=(1<<PD0)|(0<<PD1)|(0<<PD2)|(1<<PD3);
_delay_ms(50);
stepper=(0<<PD0)|(0<<PD1)|(1<<PD2)|(1<<PD3);
_delay_ms(50);
stepper=(0<<PD0)|(1<<PD1)|(1<<PD2)|(0<<PD3);
_delay_ms(50);
stepper=(1<<PD0)|(1<<PD1)|(0<<PD2)|(0<<PD3);
_delay_ms(50);
}
}
full_step_high_torque_right()
{
while(1)
{
stepper=(1<<PD0)|(1<<PD1)|(0<<PD2)|(0<<PD3);
_delay_ms(50);
stepper=(0<<PD0)|(1<<PD1)|(1<<PD2)|(0<<PD3);
_delay_ms(50);
stepper=(0<<PD0)|(0<<PD1)|(1<<PD2)|(1<<PD3);
_delay_ms(50);
stepper=(1<<PD0)|(0<<PD1)|(0<<PD2)|(1<<PD3);
_delay_ms(50);
}
}
full_step_low_torque_left()
{
while(1)
{
stepper=(1<<PD0)|(0<<PD1)|(0<<PD2)|(0<<PD3);
_delay_ms(50);
stepper=(0<<PD0)|(0<<PD1)|(0<<PD2)|(1<<PD3);
_delay_ms(50);
stepper=(0<<PD0)|(0<<PD1)|(1<<PD2)|(0<<PD3);
_delay_ms(50);
stepper=(0<<PD0)|(1<<PD1)|(0<<PD2)|(0<<PD3);
_delay_ms(50);
}
}
full_step_low_torque_right()
{
while(1)
{
stepper=(1<<PD0)|(0<<PD1)|(0<<PD2)|(0<<PD3);
_delay_ms(50);
stepper=(0<<PD0)|(1<<PD1)|(0<<PD2)|(0<<PD3);
_delay_ms(50);
stepper=(0<<PD0)|(0<<PD1)|(1<<PD2)|(0<<PD3);
_delay_ms(50);
stepper=(0<<PD0)|(0<<PD1)|(0<<PD2)|(1<<PD3);
_delay_ms(50);
}
}
half_step_left()
{
while(1)
{
stepper=(1<<PD0)|(0<<PD1)|(0<<PD2)|(0<<PD3);
_delay_ms(50);
stepper=(1<<PD0)|(0<<PD1)|(0<<PD2)|(1<<PD3);
_delay_ms(50);
stepper=(0<<PD0)|(0<<PD1)|(0<<PD2)|(1<<PD3);
_delay_ms(50);
stepper=(0<<PD0)|(0<<PD1)|(1<<PD2)|(1<<PD3);
_delay_ms(50);
stepper=(0<<PD0)|(0<<PD1)|(1<<PD2)|(0<<PD3);
_delay_ms(50);
stepper=(0<<PD0)|(1<<PD1)|(1<<PD2)|(0<<PD3);
_delay_ms(50);
stepper=(0<<PD0)|(1<<PD1)|(0<<PD2)|(0<<PD3);
_delay_ms(50);
stepper=(1<<PD0)|(1<<PD1)|(0<<PD2)|(0<<PD3);
_delay_ms(50);
}
}
half_step_right()
{
while(1)
{
stepper=(1<<PD0)|(0<<PD1)|(0<<PD2)|(0<<PD3);
_delay_ms(50);
stepper=(1<<PD0)|(1<<PD1)|(0<<PD2)|(0<<PD3);
_delay_ms(50);
stepper=(0<<PD0)|(1<<PD1)|(0<<PD2)|(0<<PD3);
_delay_ms(50);
stepper=(0<<PD0)|(1<<PD1)|(1<<PD2)|(0<<PD3);
_delay_ms(50);
stepper=(0<<PD0)|(0<<PD1)|(1<<PD2)|(0<<PD3);
_delay_ms(50);
stepper=(0<<PD0)|(0<<PD1)|(1<<PD2)|(1<<PD3);
_delay_ms(50);
stepper=(0<<PD0)|(0<<PD1)|(0<<PD2)|(1<<PD3);
_delay_ms(50);
stepper=(1<<PD0)|(0<<PD1)|(0<<PD2)|(1<<PD3);
_delay_ms(50);
}
}
stop()
{
while(1)
stepper=0x00;
}
Circuit Diagram:
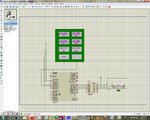