ishailesh
Junior Member level 3
- Joined
- Apr 4, 2012
- Messages
- 31
- Helped
- 6
- Reputation
- 12
- Reaction score
- 6
- Trophy points
- 1,288
- Location
- New Delhi, India
- Activity points
- 1,652
I am trying to implement a PRBS generator as show in diagram.
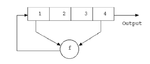
My top level module is
and dff entity is
I am using Xilinx ISE 10.1 on 64 bit Windows 7.
Now when i synthesize dff then synthesis tool returns no error.
But when i synthesize prbs then it show errors.
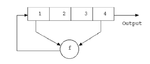
My top level module is
Code:
library IEEE;
use IEEE.STD_LOGIC_1164.ALL;
use IEEE.STD_LOGIC_ARITH.ALL;
use IEEE.STD_LOGIC_UNSIGNED.ALL;
---- Uncomment the following library declaration if instantiating
---- any Xilinx primitives in this code.
--library UNISIM;
--use UNISIM.VComponents.all;
entity prbs is
Port ( clock : in STD_LOGIC;
reset : IN std_logic := '0';
prbsout : out STD_LOGIC);
end prbs;
architecture Behavioral of prbs is
COMPONENT dff
PORT(
clock : IN std_logic;
reset : IN std_logic := '0';
din : IN std_logic := '1';
dout : OUT std_logic
);
END COMPONENT;
signal temp : std_logic := '0';
begin
genreg : for i in 0 to 3 generate
begin
instdff : entity dff port map ( clock , reset , din(i) , dout(i));
end generate genreg;
main : process(clock)
begin
for j in 0 to 3 loop
if ( j /= 0) then
din(j) <= dout(j-1);
else
din(j) <= dout(0) xor dout(2);
temp <= dout(j);
end if;
end loop;
prbsout <= temp;
end process main;
end Behavioral;
and dff entity is
Code:
library IEEE;
use IEEE.STD_LOGIC_1164.ALL;
use IEEE.STD_LOGIC_ARITH.ALL;
use IEEE.STD_LOGIC_UNSIGNED.ALL;
entity dff is
Port ( clock : in STD_LOGIC ;
reset : in STD_LOGIC := '0';
din : in STD_LOGIC := '1';
dout : out STD_LOGIC);
end dff;
architecture Behavioral of dff is
begin
process(clock)
begin
if clock'event and clock = '1' then
if reset = '0' then
-- dout <= din;
else
dout <= '0';
end if;
end if;
end process;
end Behavioral;
I am using Xilinx ISE 10.1 on 64 bit Windows 7.
Now when i synthesize dff then synthesis tool returns no error.
But when i synthesize prbs then it show errors.
ERROR:HDLParsers:3313 - "E:/IIT/modulator/source/prbs.vhd" Line 51. Undefined symbol 'din'. Should it be: in or min?
ERROR:HDLParsers:1209 - "E:/IIT/modulator/source/prbs.vhd" Line 51. din: Undefined symbol (last report in this block)
ERROR:HDLParsers:3313 - "E:/IIT/modulator/source/prbs.vhd" Line 51. Undefined symbol 'dout'. Should it be: out?
ERROR:HDLParsers:1209 - "E:/IIT/modulator/source/prbs.vhd" Line 51. dout: Undefined symbol (last report in this block)
ERROR:HDLParsers:3324 - "E:/IIT/modulator/source/prbs.vhd" Line 51. IN mode Formal din of entity with no default value must be associated with an actual value.
ERROR:HDLParsers:3313 - "E:/IIT/modulator/source/prbs.vhd" Line 59. Undefined symbol 'din'. Should it be: in or min?
ERROR:HDLParsers:1209 - "E:/IIT/modulator/source/prbs.vhd" Line 59. din: Undefined symbol (last report in this block)
ERROR:HDLParsers:3313 - "E:/IIT/modulator/source/prbs.vhd" Line 59. Undefined symbol 'dout'. Should it be: out?
ERROR:HDLParsers:1209 - "E:/IIT/modulator/source/prbs.vhd" Line 59. dout: Undefined symbol (last report in this block)
Last edited: