owenljn
Newbie level 6

- Joined
- Feb 14, 2013
- Messages
- 14
- Helped
- 0
- Reputation
- 0
- Reaction score
- 0
- Trophy points
- 1,281
- Activity points
- 1,401
Here's the question:
We wish to implement a nite state machine (FSM) that recognizes two specic sequences of applied input
symbols, namely four consecutive 1s or four consecutive 0s. There is an input w and an output z. Whenever
w = 1 or w = 0 for four consecutive clock pulses the value of z has to be 1; otherwise, z = 0. Overlapping
sequences are allowed, so that if w = 1 for ve consecutive clock pulses the output z will be equal to 1 after the
fourth and fth pulses. Figure 1 illustrates the required relationship between w and z.
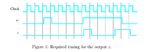
A state diagram for this FSM is shown in Figure 2.
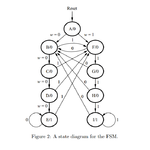
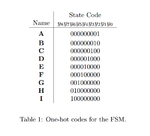
Write a Verilog file that instantiates the nine flip-flops in the circuit and which species the logic expressions
that drive the flip-flop input ports. Use only simple assign statements in your Verilog code to specify
the logic feeding the flip-flops. Note that the one-hot code enables you to derive these expressions by
inspection.
Use the toggle switch SW0 on the DE2-series board as an active-low synchronous reset input for the
FSM, use SW1 as the w input, and the pushbutton KEY0 as the clock input which is applied manually.
Use the green light LEDG0 as the output z, and assign the state flip-flop outputs to the red lights LEDR8
to LEDR0.
Here's my code, it works for transitions from A to H, but when I press clock after H, the state stay at state H and won't go to state I, can anyone help me fix this code??
We wish to implement a nite state machine (FSM) that recognizes two specic sequences of applied input
symbols, namely four consecutive 1s or four consecutive 0s. There is an input w and an output z. Whenever
w = 1 or w = 0 for four consecutive clock pulses the value of z has to be 1; otherwise, z = 0. Overlapping
sequences are allowed, so that if w = 1 for ve consecutive clock pulses the output z will be equal to 1 after the
fourth and fth pulses. Figure 1 illustrates the required relationship between w and z.
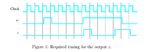
A state diagram for this FSM is shown in Figure 2.
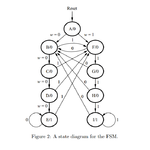
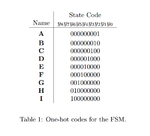
Write a Verilog file that instantiates the nine flip-flops in the circuit and which species the logic expressions
that drive the flip-flop input ports. Use only simple assign statements in your Verilog code to specify
the logic feeding the flip-flops. Note that the one-hot code enables you to derive these expressions by
inspection.
Use the toggle switch SW0 on the DE2-series board as an active-low synchronous reset input for the
FSM, use SW1 as the w input, and the pushbutton KEY0 as the clock input which is applied manually.
Use the green light LEDG0 as the output z, and assign the state flip-flop outputs to the red lights LEDR8
to LEDR0.
Here's my code, it works for transitions from A to H, but when I press clock after H, the state stay at state H and won't go to state I, can anyone help me fix this code??
Code:
module part1(SW, KEY, LEDG, LEDR);
input [1:0] KEY, SW;
output [1:0] LEDG;
output [17:0] LEDR;
reg z;
reg [3:0] shifter;
reg [8:0] Y;
always @(posedge KEY[0] or posedge SW[0]) begin
if (SW[0]) begin
z <= 1'b0;
shifter <= 4'b0101;
Y <= 9'b000000001; // A
end
else begin
if (shifter == 4'b0000)
z <= 1'b0;
else if (shifter == 4'b1111)
z <= 1'b1;
else
if ((shifter == 4'b0110)|(shifter == 4'b1110)|(shifter == 4'b0010)|((shifter == 4'b0101)&~SW[1]))
Y <= 9'b000000010; // B
else if ((shifter == 4'b1010)|(shifter == 4'b1100))
Y <= 9'b000000100; // C
else if (shifter == 4'b0100)
Y <= 9'b000001000; // D
else if ((shifter == 4'b0000)|(shifter == 4'b1000))
Y <= 9'b000010000; // E
else if ((shifter == 4'b0001)|(shifter == 4'b1101)|(shifter == 4'b1001)|((shifter == 4'b0101)&SW[1]))
Y <= 9'b000100000; // F
else if ((shifter == 4'b0011)|(shifter == 4'b1011))
Y <= 9'b001000000; // G
else if (shifter == 4'b0111)
Y <= 9'b010000000; // H
else if (shifter == 4'b1111)
Y <= 9'b100000000; // I
shifter[3:0] <= { shifter[2:0] , SW[1] };
end
end
assign LEDG[0] = z;
assign LEDR[8:0] = Y;
assign LEDR[17:14] = shifter;
endmodule
Last edited: