xpress_embedo
Advanced Member level 4
Hello!! Everyone,
I am using ATtiny85 micro-controller and have to Sniff the I2C bus and watch its data on UART.
I found one example on AVR freaks website
https://www.avrfreaks.net/projects/i2c-twi-sniffer
This works perfectly but the problem is that, it uses USI hardware, and i can't use that, in my hardware PB1 and PB4 pins are used to sniff the I2C data.
PB1 will Sniff SCL line and PB4 will Sniff SDA line.
I don't know, why i am not able to detect the start condition, with this code can any one please help me in this.
I am using PIC16F877A and DS1307 both communication with each other, and using ATtiny85 to sniff its data.
Hardware
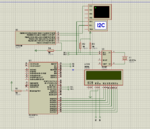
Simulation
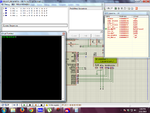
Please suggest me what i am doing wrong.
I am using ATtiny85 micro-controller and have to Sniff the I2C bus and watch its data on UART.
I found one example on AVR freaks website
https://www.avrfreaks.net/projects/i2c-twi-sniffer
This works perfectly but the problem is that, it uses USI hardware, and i can't use that, in my hardware PB1 and PB4 pins are used to sniff the I2C data.
PB1 will Sniff SCL line and PB4 will Sniff SDA line.
I don't know, why i am not able to detect the start condition, with this code can any one please help me in this.
Code C - [expand] 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 #define SCL_SNIFF 1 #define SDA_SNIFF 4 #define IDLE 0 #define START 1 #define DATA 2 #define STOP 3 #define INVAILD 4 #define LOW 0 #define HIGH (!LOW) char state = IDLE; char sda_state = LOW, sda_state_prev = HIGH; char scl_state = LOW, scl_state_prev = HIGH; char readPin(char PinNumber) { char status = LOW; if(PINB&(1<<PinNumber)) { status = HIGH; } return status; } int main( void ) { // Enable Internal Pull-Ups PORTB |= (1<<SCL_SNIFF); PORTB |= (1<<SDA_SNIFF); // Make Pins as Input Output Pins DDRB &= ~(1<<SCL_SNIFF); DDRB &= ~(1<<SDA_SNIFF); init_uart0(); sei(); uputs0( "I2C-Sniffer:\n"); while(1) { scl_state = readPin(SCL_SNIFF); sda_state = readPin(SDA_SNIFF); if(scl_state != scl_state_prev) { scl_state_prev = scl_state; } if(sda_state != sda_state_prev) { sda_state_prev = sda_state; } switch(state) { case IDLE: if(scl_state == HIGH) { if((sda_state_prev == HIGH) & (sda_state == LOW)) { state = START; } if((sda_state_prev == LOW) & (sda_state == HIGH)) { state = STOP; } } break; case START: uputs0( "Start"); state = IDLE; break; case DATA: break; case STOP: uputs0( "Stop\n"); state = IDLE; break; case INVAILD: default: state = IDLE; break; } } }
I am using PIC16F877A and DS1307 both communication with each other, and using ATtiny85 to sniff its data.
Hardware
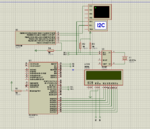
Simulation
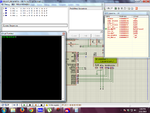
Please suggest me what i am doing wrong.