bitprolix
Junior Member level 2

- Joined
- Sep 19, 2013
- Messages
- 24
- Helped
- 0
- Reputation
- 0
- Reaction score
- 0
- Trophy points
- 1
- Activity points
- 235
Hello everyone,
After some pointers from some expert from this forum for my up-down VHDL counter, I was able to successfully design such a counter. For testing my design, I was given a test bench. My design looks fine, and after looking at the timing diagram, I can see that there is a 2 clock tick delay in my counter, but I'm not able to figure out what is the exact instruction in the testbench, that is causing that delay. I see there are couple of "wait" instruction below. Without understanding properly, what is causing that delay, I started changing some wait instructions below, and started getting more weird waveforms. This is indeed incorrect way to understand what's going on here. Therefore, Can someone please throw some light here. If you look at the waveform attached below, you'll find a 2clock cycle delay when the counter is counting down. It does the counting at the rising edge and only if the ENABLE bit is set, but, I see that at ~380ns, though the ENABLE bit is set, the counter doesn't start counting, until next clock tick. And because of this it is taking 2 additional clock ticks to count from 13 to 5. Why so? For reference purpose, I've put my design, the testbech code, and the resulting waveform below.
1: The counter design
2: The testbench (not written by me)
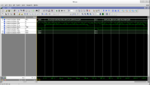
After some pointers from some expert from this forum for my up-down VHDL counter, I was able to successfully design such a counter. For testing my design, I was given a test bench. My design looks fine, and after looking at the timing diagram, I can see that there is a 2 clock tick delay in my counter, but I'm not able to figure out what is the exact instruction in the testbench, that is causing that delay. I see there are couple of "wait" instruction below. Without understanding properly, what is causing that delay, I started changing some wait instructions below, and started getting more weird waveforms. This is indeed incorrect way to understand what's going on here. Therefore, Can someone please throw some light here. If you look at the waveform attached below, you'll find a 2clock cycle delay when the counter is counting down. It does the counting at the rising edge and only if the ENABLE bit is set, but, I see that at ~380ns, though the ENABLE bit is set, the counter doesn't start counting, until next clock tick. And because of this it is taking 2 additional clock ticks to count from 13 to 5. Why so? For reference purpose, I've put my design, the testbech code, and the resulting waveform below.
1: The counter design
Code:
--Counter design
library IEEE;
use IEEE.std_logic_1164.all;
use IEEE.std_logic_arith.all;
--Entity
entity Counter is
generic ( BIT_WIDTH : positive );
port (
CLK : in bit;
RESET, ENABLE : in std_logic;
STARTADR, STOPADR : in unsigned ( BIT_WIDTH - 1 downto 0 );
ADDRESS : out unsigned ( BIT_WIDTH - 1 downto 0) );
end Counter;
--Register-tranfer level
architecture RTL of Counter is
signal counter : unsigned ( BIT_WIDTH -1 downto 0 );
begin
Cnt: process(CLK, RESET)
begin
if ( RESET = '1' ) then
counter <= STARTADR;
elsif (CLK='1' and CLK'event ) then
if ( ENABLE = '1' ) and ( counter < STOPADR ) then
counter <= counter + 1;
elsif ( ENABLE = '1' ) and ( counter > STOPADR ) then
counter <= counter - 1;
end if;
end if;
end process Cnt;
-- A signal assignment doesn't happen immediately, unlike variable
-- assignment. It happens at some later time.
ADDRESS <= counter;
end RTL;
2: The testbench (not written by me)
Code:
--Counter testbench
entity CounterTestBench is
end CounterTestBench;
--Content of the environment
library IEEE;
use IEEE.std_logic_1164.all;
use IEEE.std_logic_arith.all;
architecture Bench of CounterTestBench is
constant BW : integer := 4;
signal Clk : bit := '0';
signal Rst, Ena : std_logic := '0';
signal Strt, Stp, Addr : unsigned ( BW - 1 downto 0 ) := ( others => '0' );
signal Halt : boolean := false;
--Unit Under Test (UUT)
component Counter
generic ( BIT_WIDTH : positive );
port (
CLK : in bit;
RESET, ENABLE : in std_logic;
STARTADR, STOPADR : in unsigned ( BIT_WIDTH - 1 downto 0 );
ADDRESS : out unsigned ( BIT_WIDTH - 1 downto 0 ) );
end component;
begin
--UUT
UUT: Counter generic map (BW) port map ( Clk, Rst, Ena, Strt, Stp, Addr );
--Clock generator
Clk <= not Clk after 10 ns when not Halt else unaffected;
--Test sequence
Test_sequence: process
procedure MakeSequence
( constant START_VALUE, STOP_VALUE, CYCLES_COUNT : positive ) is
begin
wait on Clk until Clk = '1';
Strt <= conv_unsigned ( START_VALUE, BW ) after 1 ns;
Stp <= conv_unsigned ( STOP_VALUE, BW ) after 1 ns;
Rst <= '1' after 1 ns;
Ena <= '0' after 1 ns;
wait on Clk until Clk = '1';
Rst <= '0' after 1 ns;
wait on Clk until Clk = '1';
Ena <= '1' after 1 ns;
for i in 0 to CYCLES_COUNT - 4 loop
wait on Clk until Clk = '1';
end loop;
Ena <= '0' after 1 ns;
wait on Clk until Clk = '1';
end MakeSequence;
begin
MakeSequence ( 2, 12, 16 ); --From 2 to 12, 16 cycles
MakeSequence ( 13, 5, 16 ); --From 13 down to 5, 16 cycles
Strt <= ( others => '0' ) after 1 ns;
Stp <= ( others => '0' ) after 1 ns;
Halt <= true after 50 ns;
wait;
end process Test_sequence;
end Bench;
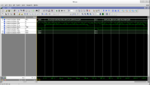