ADGAN
Full Member level 5
Hi! I'm doing a small project as a hobby which displays time in a big font and date. I'm using DS1307, PIC18F45K22 and 20x4 LCD. The clock is working fine. But I have some issues when displaying the time using a big font. Some digits are not displaying properly. Also I want to display time in 12 hr mode and display AM/PM depending on the time. I'm bit stuck on how to do it.
The header file which includes the big fonts
This is how 10:13 is displayed
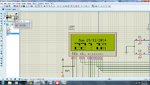
Code:
#include "customChars.h"
// LCD module connections
sbit LCD_RS at RB2_bit;
sbit LCD_EN at RB3_bit;
sbit LCD_D4 at RB4_bit;
sbit LCD_D5 at RB5_bit;
sbit LCD_D6 at RB6_bit;
sbit LCD_D7 at RB7_bit;
sbit LCD_RS_Direction at TRISB2_bit;
sbit LCD_EN_Direction at TRISB3_bit;
sbit LCD_D4_Direction at TRISB4_bit;
sbit LCD_D5_Direction at TRISB5_bit;
sbit LCD_D6_Direction at TRISB6_bit;
sbit LCD_D7_Direction at TRISB7_bit;
// End LCD module connections
unsigned char txt1[] = "Hello";
unsigned char txt2[] = "20";
unsigned char txt3[] = "AM";
unsigned char txt4[] = "PM";
unsigned char txt[14];
/*******************************************************************************
* DS1307 Functions
*******************************************************************************/
// RTC Definitions
#define RTC_ADDR 0xD0
// Global date/time variables
unsigned short int seconds, minutes, hours, day, week, month, year;
/*******************************************************************************
* Read time from RTC DS1307
* input : pointer to variables where RTC data will be stored
* output: variables with RTC data
*******************************************************************************/
void Read_Time(unsigned short int *hours, unsigned short int *minutes,
unsigned short int *seconds,unsigned short int *day, unsigned short int *week,
unsigned short int *month, unsigned short int *year){
I2C1_Start(); // Issue start signal
I2C1_Wr(RTC_ADDR); // Address DS1307, see DS1307 datasheet
I2C1_Wr(0); // Start from address 0
I2C1_Repeated_Start(); // Issue repeated start signal
I2C1_Wr(RTC_ADDR + 1); // Address DS1307 for reading R/W=1
*seconds = I2C1_Rd(1); // Read seconds byte
*minutes = I2C1_Rd(1); // Read minutes byte
*hours = I2C1_Rd(1); // Read hours byte
*week =I2C1_Rd(1); // Read week day byte
*day =I2C1_Rd(1); // Read day byte
*month =I2C1_Rd(1); // Read month byte
*year =I2C1_Rd(0); // Read year byte
I2C1_Stop(); // Issue stop signal
}
/*******************************************************************************
* Write time to RTC DS1307
* input : variables with RTC data
*******************************************************************************/
void Write_Time(unsigned short int hours, unsigned short int minutes,
unsigned short int seconds,unsigned short int day, unsigned short int week,
unsigned short int month, unsigned short int year){
unsigned char tmp1, tmp2;
tmp1 = minutes / 10; //Write tens of minute
tmp2 = minutes % 10; //Write unit of minute
minutes = tmp1 * 16 + tmp2; //Includes all value
tmp1 = hours / 10; //Write tens of hour
tmp2 = hours % 10; //Write unit of hour
hours = tmp1 * 16 + tmp2; //Includes all value
tmp1 = week / 10; //Write tens of weekday
tmp2 = week % 10; //Write unit of weekday
week = tmp1 *16 +tmp2; //Includes all value
tmp1 = day / 10; //Write tens of day
tmp2 = day % 10; //Write unit of day
day = tmp1 *16 +tmp2; //Includes all value
tmp1 = month / 10; //Write tens of month
tmp2 = month % 10; //Write unit of month
month = tmp1 *16 +tmp2; //Includes all value
tmp1 = year / 10; //Write tens of year
tmp2 = year % 10; //Write unit of year
year = tmp1 *16 +tmp2; //Includes all value
I2C1_Start(); // issue start signal
I2C1_Wr(RTC_ADDR); // address DS1307
I2C1_Wr(0); // start from word at address (REG0)
I2C1_Wr(0x80); // write $80 to REG0. (pause counter + 0 sec)
I2C1_Wr(minutes); // write 0 to minutes word to (REG1)
I2C1_Wr(hours); // write 17 to hours word (24-hours mode)(REG2)
I2C1_Wr(week); // write 2 - Monday (REG3)
I2C1_Wr(day); // write 4 to date word (REG4)
I2C1_Wr(month); // write 5 (May) to month word (REG5)
I2C1_Wr(year); // write 01 to year word (REG6)
I2C1_Stop(); // issue stop signal
I2C1_Start(); // issue start signal
I2C1_Wr(RTC_ADDR); // address DS1307
I2C1_Wr(0); // start from word at address 0
I2C1_Wr(0 | seconds); // write 0 to REG0 (enable counting + 0 sec)
I2C1_Stop(); // issue stop signal
}
unsigned char h1,h2,s1,s2;
unsigned char Dsec;
/*******************************************************************************
* Show on the LCD display
* input : variables with RTC data
*******************************************************************************/
void Show_Time(){
char *txt;
if(hours & 0x20 == 0x20)Lcd_out(3,16,txt4);
else Lcd_out(3,16,txt3);
//Converts variables in BCD format to Decimal
seconds = ((seconds & 0x70) >> 4)*10 + (seconds & 0x0F); //seconds
minutes = ((minutes & 0xF0) >> 4)*10 + (minutes & 0x0F); //minutes
hours = ((hours & 0x30) >> 4)*10 + (hours & 0x0F); //hours
week = (week & 0x07); //week
day = ((day & 0xF0) >> 4)*10 + (day & 0x0F); //day
month = ((month & 0x10) >> 4)*10 + (month & 0x0F); //month
year = ((year & 0xF0)>>4)*10+(year & 0x0F); //year
switch(week)
{
case 1: txt="Sun"; break;
case 2: txt="Mon"; break;
case 3: txt="Tue"; break;
case 4: txt="Wed"; break;
case 5: txt="Thu"; break;
case 6: txt="Fri"; break;
case 7: txt="Sat"; break;
}
Lcd_Out(2,4,txt); //Display the current day
Lcd_Chr(2, 8, (day / 10) + 48); //Print tens digit of day variable
Lcd_Chr(2, 9, (day % 10) + 48); //Print ones digit of day variable
Lcd_Chr_CP('/'); //Print '/' character on cursor position
Lcd_Chr(2, 11, (month / 10) + 48); //Print tens digit of month variable
Lcd_Chr(2,12, (month % 10) + 48); //Print oness digit of month variable
Lcd_Chr_CP('/'); //Print '/' character on cursor position
Lcd_Chr(2,16, (year / 10) + 48); //Print tenss digit of year variable
Lcd_Chr(2,17, (year % 10) + 48); //Print oness digit of year variable
h1 = hours/10;
h2 = hours%10;
s1 = minutes/10;
s2 = minutes%10;
switch(h1){
case 0:zero(3,2);break;
case 1:one(3,2);break;
case 2:two(3,2);break;
case 3:three(3,2);break;
case 4:four(3,2);break;
case 5:five(3,2);break;
case 6:six(3,2);break;
case 7:seven(3,2);break;
case 8:eight(3,2);break;
case 9:nine(3,2);break;
Delay_ms(100);
}
switch(h2){
case 0:zero(3,5);break;
case 1:one(3,5);break;
case 2:two(3,5);break;
case 3:three(3,5);break;
case 4:four(3,5);break;
case 5:five(3,5);break;
case 6:six(3,5);break;
case 7:seven(3,5);break;
case 8:eight(3,5);break;
case 9:nine(3,5);break;
Delay_ms(100);
}
switch(s1){
case 0:zero(3,9);break;
case 1:one(3,9);break;
case 2:two(3,9);break;
case 3:three(3,9);break;
case 4:four(3,9);break;
case 5:five(3,9);break;
case 6:six(3,9);break;
case 7:seven(3,9);break;
case 8:eight(3,9);break;
case 9:nine(3,9);break;
Delay_ms(100);
}
switch(s2){
case 0:zero(3,13);break;
case 1:one(3,13);break;
case 2:two(3,13);break;
case 3:three(3,13);break;
case 4:four(3,13);break;
case 5:five(3,13);break;
case 6:six(3,13);break;
case 7:seven(3,13);break;
case 8:eight(3,13);break;
case 9:nine(3,13);break;
Delay_ms(100);
}
Lcd_Chr(4,16, (seconds / 10) + 48); //Print tenss digit of year variable
Lcd_Chr(4,17, (seconds % 10) + 48); //Print oness digit of year variable
}
/*******************************************************************************
* Main function
*******************************************************************************/
void main()
{
ANSELA = 0; // Configure PORTA pins as digital
ANSELB = 0; // Configure PORTB pins as digital
ANSELC = 0; // Configure PORTC pins as digital
ANSELE = 0; // Configure PORTE pins as digital
SLRCON = 0; // Configure all PORTS at the standard Slew
ADCON1 |= 0x0F; // Configure AN pins as digital
C1ON_bit = 0; // Disable comparators
C2ON_bit = 0; //
TRISA = 0; //PortA configured as output
PORTA = 0;
LATA = 0;
TRISB = 0; //PortB confgured as output
PORTB = 0; //Reset PORTB
LATB = 0;
TRISC = 0x18; //T1CKI, SCL, SDA and RX set as input
PORTC = 0; //Reset PORTC
TRISD = 0;
PORTD = 0;
LATC = 0;
TRISE = 0xFF;
LATE = 0;
PORTE = 0;
Lcd_Init(); //Initialize the LCD
Delay_ms(100); // Delay 100ms
Lcd_Cmd(_LCD_CURSOR_OFF);//Cursor off
Lcd_Cmd(_LCD_CLEAR); //Clear the LCD
I2C1_Init(100000); //Initialize at 100KHz
Delay_ms (100); //Delay 100ms
LCD_Out(1,5,txt1);
LCD_Out(2,14,txt2);
while(1){
Read_Time(&hours, &minutes, &seconds, &day, &week, &month, &year);
Show_Time();
Delay_ms(100);
LATD.F3 = 1;
LATD.F4 = 1;
Delay_ms(100);
}
The header file which includes the big fonts
Code:
char i;
const char character0_0[] = {7,15,31,31,31,31,31,31};
const char character0_1[] = {31,31,0,0,0,0,0,0};
const char character0_2[] = {28,30,31,31,31,31,31,31};
const char character0_3[] = {31,31,31,31,31,31,30,28};
const char character0_4[] = {0,0,0,0,0,0,31,31};
const char character0_5[] = {31,31,31,31,31,31,15,7};
const char character1_2[] = {31,31,31,31,31,31,31,31};
const char character5_1[] = {31,31,0,0,0,0,31,31};
const char character5_4[] = {31,0,0,0,0,0,31,31};
const char character8_0[] = {7,15,30,28,30,31,15,7};
const char character8_1[] = {31,31,0,0,0,0,0,31};
const char character8_2[] = {28,30,15,7,15,31,30,28};
const char character8_3[] = {28,30,15,7,15,31,30,28};
const char character8_4[] = {31,0,0,0,0,0,31,31};
const char character8_5[] = {7,15,30,28,30,31,15,7};
const char character9_4[] = {31,0,0,0,0,0,0,0};
void zero(char pos_row, char pos_char) {
Lcd_Cmd(64);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character0_0[i]);
LCD_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row, pos_char, 0);
Lcd_Cmd(72);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character0_1[i]);
LCD_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row, pos_char+1, 1);
Lcd_Cmd(80);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character0_2[i]);
LCD_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row, pos_char+2, 2);
Lcd_Cmd(88);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character0_3[i]);
//LCD_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row+1, pos_char+2, 3);
Lcd_Cmd(96);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character0_4[i]);
// LCD_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row+1, pos_char+1, 4);
Lcd_Cmd(104);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character0_5[i]);
// LCD_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row+1, pos_char, 5);
}
void one(char pos_row, char pos_char){
Lcd_Cmd(64);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character0_1[i]);
Lcd_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row, pos_char, 0);
Lcd_Cmd(72);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character0_2[i]);
Lcd_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row, pos_char+1, 1);
Lcd_Cmd(80);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character1_2[i]);
Lcd_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row+1, pos_char+1, 2);
}
void two(char pos_row, char pos_char){
Lcd_Cmd(64);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character0_1[i]);
LCD_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row, pos_char, 0);
Lcd_Cmd(64);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character0_1[i]);
LCD_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row, pos_char+1, 0);
Lcd_Cmd(72);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character0_2[i]);
LCD_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row, pos_char+2, 1);
Lcd_Cmd(80);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character5_4[i]);
LCD_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row+1, pos_char+2, 2);
Lcd_Cmd(80);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character5_4[i]);
LCD_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row+1, pos_char+1, 2);
Lcd_Cmd(88);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character1_2[i]);
LCD_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row+1, pos_char, 3);
}
void three(char pos_row, char pos_char){
Lcd_Cmd(64);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character5_4[i]);
Lcd_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row, pos_char, 0);
Lcd_Cmd(64);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character5_4[i]);
Lcd_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row, pos_char+1, 0);
Lcd_Cmd(72);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character0_2[i]);
Lcd_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row, pos_char+2, 1);
Lcd_Cmd(80);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character0_3[i]);
Lcd_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row+1, pos_char+2, 2);
Lcd_Cmd(88);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character8_4[i]);
Lcd_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row+1, pos_char+1, 3);
Lcd_Cmd(88);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character8_4[i]);
Lcd_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row+1, pos_char, 3);
}
void four(char pos_row, char pos_char){
Lcd_Cmd(64);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character0_5[i]);
Lcd_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row, pos_char, 0);
Lcd_Cmd(72);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character0_4[i]);
Lcd_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row, pos_char+1, 1);
Lcd_Cmd(80);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character0_2[i]);
Lcd_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row, pos_char+2, 2);
Lcd_Cmd(88);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character1_2[i]);
Lcd_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row+1, pos_char+2, 3);
}
void five(char pos_row, char pos_char){
Lcd_Cmd(64);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character1_2[i]);
Lcd_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row, pos_char, 0);
Lcd_Cmd(72);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character5_1[i]);
Lcd_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row, pos_char+1, 1);
Lcd_Cmd(72);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character5_1[i]);
Lcd_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row, pos_char+2, 1);
Lcd_Cmd(80);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character0_3[i]);
Lcd_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row+1, pos_char+2, 2);
Lcd_Cmd(88);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character5_4[i]);
Lcd_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row+1, pos_char+1, 3);
Lcd_Cmd(88);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character5_4[i]);
Lcd_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row+1, pos_char, 3);
}
void six(char pos_row, char pos_char){
Lcd_Cmd(64);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character0_0[i]);
Lcd_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row, pos_char, 0);
Lcd_Cmd(72);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character8_1[i]);
Lcd_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row, pos_char+1, 1);
Lcd_Cmd(80);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character0_1[i]);
Lcd_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row, pos_char+2, 2);
Lcd_Cmd(88);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character0_3[i]);
Lcd_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row+1, pos_char+2, 3);
Lcd_Cmd(96);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character8_4[i]);
Lcd_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row+1, pos_char+1, 4);
Lcd_Cmd(104);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character0_5[i]);
Lcd_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row+1, pos_char, 5);
}
void seven(char pos_row, char pos_char){
Lcd_Cmd(64);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character0_1[i]);
Lcd_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row, pos_char, 0);
Lcd_Cmd(64);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character0_1[i]);
Lcd_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row, pos_char+1, 0);
Lcd_Cmd(72);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character0_2[i]);
Lcd_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row, pos_char+2, 1);
Lcd_Cmd(80);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character0_0[i]);
Lcd_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row+1, pos_char+1, 2);
}
void eight(char pos_row, char pos_char){
Lcd_Cmd(64);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character8_0[i]);
Lcd_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row, pos_char, 0);
Lcd_Cmd(72);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character8_1[i]);
Lcd_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row, pos_char+1, 1);
Lcd_Cmd(80);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character8_2[i]);
Lcd_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row, pos_char+2, 2);
Lcd_Cmd(88);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character8_3[i]);
Lcd_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row+1, pos_char+2, 3);
Lcd_Cmd(96);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character8_4[i]);
Lcd_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row+1, pos_char+1, 4);
Lcd_Cmd(104);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character8_5[i]);
Lcd_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row+1, pos_char, 5);
}
void nine(char pos_row, char pos_char){
Lcd_Cmd(64);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character0_0[i]);
Lcd_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row, pos_char, 0);
Lcd_Cmd(72);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character8_1[i]);
Lcd_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row, pos_char+1, 1);
Lcd_Cmd(80);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character0_2[i]);
Lcd_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row, pos_char+2, 2);
Lcd_Cmd(88);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character1_2[i]);
Lcd_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row+1, pos_char+2, 3);
Lcd_Cmd(96);
for (i = 0; i<=7; i++) Lcd_Chr_CP(character9_4[i]);
Lcd_Cmd(_LCD_RETURN_HOME);
Lcd_Chr(pos_row+1, pos_char+1, 4);
}
This is how 10:13 is displayed
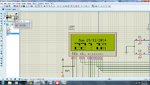