milan.km
Member level 3
hi
i wrote the following code for makin a block ram,but as my input is 32 bit width,32 DFFs inferred too,
is this sth wrong with my code?
single port block ram
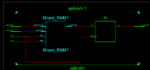
i wrote the following code for makin a block ram,but as my input is 32 bit width,32 DFFs inferred too,
is this sth wrong with my code?
single port block ram
Code:
library ieee;
use ieee.std_logic_1164.all;
use ieee.std_logic_unsigned.all;
use ieee.std_logic_arith.all;
entity spbram is
generic ( w : integer := 32;
-- number of bits per RAM word
r : integer := 12);
-- 2^r = number of words in RAM
port (clk : in std_logic;
we : in std_logic;
adr : in std_logic_vector(r-1 downto 0);
di : in std_logic_vector(w-1 downto 0);
do : out std_logic_vector(w-1 downto 0));
end spbram;
architecture spbram_arch of spbram is
type ram_type is array (2**r-1 downto 0) of std_logic_vector (w-1 downto 0);
signal RAM : ram_type;
begin
process (clk)
begin
if (clk'event and clk = '1') then
if (we = '1') then
RAM(conv_integer(unsigned(adr))) <= di;
end if;
do <= RAM(conv_integer(unsigned(adr)));
end if;
end process;
end spbram_arch;
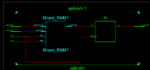