gavingt
Newbie

- Joined
- Dec 9, 2013
- Messages
- 3
- Helped
- 0
- Reputation
- 0
- Reaction score
- 0
- Trophy points
- 1,281
- Activity points
- 1,350
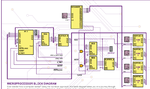
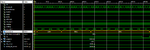
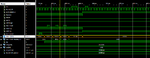
I'm getting this warning: Xst:2040 - Unit Microprocessor: 4 multi-source signals are replaced by logic (pull-up yes): A<0>, A<1>, A<2>, A<3>, but I don't know if that's a standard thing that Xilinx does or not. A(3:0) is the main bus going from the ROMs to the ALU.
I'm just trying to pass values through the ALU right now. As you can see in attached waveform, it's replacing every '0' with 'U's, but leaving the '1's intact (compare Aluout and hex_input_display).
Oh, and in addition to the components pictured, I made a somewhat sloppy display decoder for displaying results and debouncing two of the push button inputs.
Here's the overall VHDL file:
Code VHDL - [expand] 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 library IEEE; use IEEE.STD_LOGIC_1164.ALL; entity Microprocessor is Port ( clk : in STD_LOGIC; -- user-operated clock board_clk : in STD_LOGIC; -- clock from FPGA board Acctodatabus : in STD_LOGIC; Loadacc : in STD_LOGIC := '1'; Invert : in STD_LOGIC; Aonly : in STD_LOGIC; Arithlogic : in STD_LOGIC; hex_input : in STD_LOGIC; reading : in STD_LOGIC := '1'; writing : in STD_LOGIC := '0'; rom_select : in STD_LOGIC := '0'; Aluout : out STD_LOGIC_VECTOR (3 downto 0); hex_input_display : out STD_LOGIC_VECTOR (3 downto 0); DP : out STD_LOGIC; SSEG : out STD_LOGIC_VECTOR (6 downto 0); AN : out STD_LOGIC_VECTOR (3 downto 0) ); end Microprocessor; architecture Structural of Microprocessor is Component ALU is Port ( A : in STD_LOGIC_VECTOR (3 downto 0); B : in STD_LOGIC_VECTOR (3 downto 0); Cin : in STD_LOGIC; Arithlogic : in STD_LOGIC; Invert : in STD_LOGIC; Aonly : in STD_LOGIC; Y : out STD_LOGIC_VECTOR (3 downto 0); Cout : out STD_LOGIC ); end component ALU; Component REG4 is Port ( A : in STD_LOGIC_VECTOR (3 downto 0); IE : in STD_LOGIC; CLK : in STD_LOGIC; CLR : in STD_LOGIC; Y : out STD_LOGIC_VECTOR (3 downto 0) ); end component REG4; Component DECODER_4 is Port ( A : in STD_LOGIC_VECTOR (3 downto 0); EN : in STD_LOGIC; YF : out STD_LOGIC; YE : out STD_LOGIC; YD : out STD_LOGIC; YC : out STD_LOGIC; YB : out STD_LOGIC; YA : out STD_LOGIC; Y9 : out STD_LOGIC; Y8 : out STD_LOGIC; Y7 : out STD_LOGIC; Y6 : out STD_LOGIC; Y5 : out STD_LOGIC; Y4 : out STD_LOGIC; Y3 : out STD_LOGIC; Y2 : out STD_LOGIC; Y1 : out STD_LOGIC; Y0 : out STD_LOGIC); end component DECODER_4; Component BUFFER4 is Port ( EN1 : in STD_LOGIC; EN2 : in STD_LOGIC; A : in STD_LOGIC_VECTOR (3 downto 0); Y : out STD_LOGIC_VECTOR (3 downto 0) ); end Component BUFFER4; Component display_controller is Port ( board_clk : in STD_LOGIC; hex_input : in STD_LOGIC; --BTN0 for selecting hex input value rom_select : in STD_LOGIC; --BTN1 for selecting ROM Regout : in STD_LOGIC_VECTOR (3 downto 0); --receives final output from register SSEG : out STD_LOGIC_VECTOR (6 downto 0); rom_selected : out STD_LOGIC_VECTOR (3 downto 0); DP: out STD_LOGIC; -- decimal point light indicates ROM 4 selected AN : out STD_LOGIC_VECTOR (3 downto 0); hex_input_display : out STD_LOGIC_VECTOR (3 downto 0)); end component display_controller; signal A : STD_LOGIC_VECTOR (3 downto 0):= (others => '0'); -- main bus line A signal B : STD_LOGIC_VECTOR (3 downto 0):= (others => '0'); -- B bus signal and1out, and2out : STD_LOGIC; signal Alu_to_Reg4_1 : STD_LOGIC_VECTOR (3 downto 0) := (others => '0'); -- connects ALU to Reg4_1 signal Reg4_2out : STD_LOGIC_VECTOR (3 downto 0); -- leaves outputs register, goes into display_controller signal Reg4_3_to_Buffer_2 : STD_LOGIC_VECTOR (3 downto 0); -- connects Reg4_3 to Buffer_2 signal DECODER_4_out0 : STD_LOGIC; -- output 0 of decoder_4 signal DECODER_4_out1 : STD_LOGIC; -- output 1 of decoder_4 signal DECODER_4_out2 : STD_LOGIC; -- output 2 of decoder_4 signal DECODER_4_out3 : STD_LOGIC; -- output 3 of decoder_4 signal DECODER_4_outF : STD_LOGIC; -- output signal hex_input_display_signal : STD_LOGIC_VECTOR (3 downto 0) := (others => '0'); signal rom_selected_signal : STD_LOGIC_VECTOR (3 downto 0); begin hex_input_display <= hex_input_display_signal; Aluout <= Alu_to_Reg4_1; and1out <= DECODER_4_out0 and writing; and2out <= writing and DECODER_4_outF; ALU_1: ALU port map (A => A, B => B, Cin => '0', Arithlogic => Arithlogic, Invert => Invert, Aonly => Aonly, Y => Alu_to_Reg4_1); REG4_1: REG4 port map (A => Alu_to_Reg4_1, IE => Loadacc, CLK => clk, CLR => '0', Y => B); REG4_2: REG4 port map (A => A, IE => and1out, CLK => clk, CLR => '0', Y => Reg4_2out); REG4_3: REG4 port map (A => A, IE => and2out, CLK => clk, CLR => '0', Y => Reg4_3_to_Buffer_2); display_controller_1: display_controller port map(board_clk => board_clk, hex_input => hex_input, rom_select => rom_select, Regout => Reg4_2out, SSEG => SSEG, rom_selected => rom_selected_signal, DP => DP, AN => AN, hex_input_display => hex_input_display_signal); BUFFER4_1: BUFFER4 port map (EN1 => Acctodatabus, EN2 => Acctodatabus, A => B, Y => A); BUFFER4_2: BUFFER4 port map (EN1 => reading, EN2 => DECODER_4_outF, A => Reg4_3_to_Buffer_2, Y => A); BUFFER4_3: BUFFER4 port map (EN1 => DECODER_4_out0, EN2 => reading, A => hex_input_display_signal, Y => A); BUFFER4_4: BUFFER4 port map (EN1 => DECODER_4_out1, EN2 => reading, A => hex_input_display_signal, Y => A); BUFFER4_5: BUFFER4 port map (EN1 => DECODER_4_out2, EN2 => reading, A => hex_input_display_signal, Y => A); BUFFER4_6: BUFFER4 port map (EN1 => DECODER_4_out3, EN2 => reading, A => hex_input_display_signal, Y => A); DECODER_4_1: DECODER_4 port map (A => rom_selected_signal, EN => '1', YF => DECODER_4_outF, Y3 => DECODER_4_out3, Y2 => DECODER_4_out2, Y1 => DECODER_4_out1, Y0 => DECODER_4_out0); end Structural;
Last edited by a moderator: