hbelkasmi
Newbie
hello,
I'm trying to implement a 32 bit ALU with Input a,b and output Result and 4 bit ALUFlags in system verilog.
the problem for me is that i Don't know how to implement the ALUFlags especially the Carryout.
should I use the one bit adder/sabstract .
Could any one help me
thank you in advance
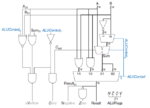
this my code
I'm trying to implement a 32 bit ALU with Input a,b and output Result and 4 bit ALUFlags in system verilog.
the problem for me is that i Don't know how to implement the ALUFlags especially the Carryout.
should I use the one bit adder/sabstract .
Could any one help me
thank you in advance
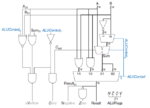
this my code
Code Verilog - [expand] 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 module Homework_4( input [31:0] a,b, // ALU 32-bit Inputs input [1:0] ALUControl,// ALU Control output [31:0] Result, // ALU 32-bit Output output [4:0] ALUFlags // ); reg [31:0] Result; always_comb begin case(ALUControl) 2'b00: // Addition Result = a + b ; 2'b01: // Subtraction assign b = ~b+1 Result = a + b ; 2'b10: // Logical and Result = a & b; 2'b11: // Logical or Result = a | b; default: Result == 0 ; endcase end endmodule
Last edited by a moderator: