FecP
Newbie level 6
This is code to detect falling pulses from two inputs.As the image attached shows, as soon as v_sync_a goes low or v_sync_b goes low, v_sync_a_falling goes or v_sync_b_falling go high. Now, let's say that the code were run on Hardware, If v_sync_a or v_sync_b fall at the exact same instant as the rising edge of the clock.How can the FPGA (Spartan-3E) operate as it does? Aren't there setup and hold time violations here?
Test Bench :
Does this code run in hardware as it does in simulation? If so, how?
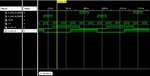
Code:
module question(
input reset,
input clk,
input v_sync_a,
input v_sync_b,
output reg v_sync_a_falling,
output reg v_sync_b_falling
);
reg v_sync_a_r;
reg v_sync_b_r;
always @(posedge clk)
begin
if(reset)
begin
v_sync_a_r <= 0;
v_sync_b_r <= 0;
end
else
begin
v_sync_a_r <= v_sync_a;
v_sync_b_r <= v_sync_b;
v_sync_a_falling <= (v_sync_a < v_sync_a_r) ? 1 : 0;
v_sync_b_falling <= (v_sync_b < v_sync_b_r) ? 1 : 0;
end
end
endmodule
Test Bench :
Code:
module test;
// Inputs
reg reset;
reg clk;
reg v_sync_a;
reg v_sync_b;
// Outputs
wire v_sync_a_falling;
wire v_sync_b_falling;
// Instantiate the Unit Under Test (UUT)
question uut (
.reset(reset),
.clk(clk),
.v_sync_a(v_sync_a),
.v_sync_b(v_sync_b),
.v_sync_a_falling(v_sync_a_falling),
.v_sync_b_falling(v_sync_b_falling)
);
initial begin
// Initialize Inputs
clk = 0;
v_sync_a = 1;
v_sync_b = 1;
reset = 1;
#5 reset=0;
// Wait 100 ns for global reset to finish
#100;
// Add stimulus here
end
always
#5 clk <= ~ clk;
always @ (posedge clk)
begin
#10 v_sync_a <= ~ v_sync_a;
#20 v_sync_b <= ~ v_sync_b;
end
endmodule
Does this code run in hardware as it does in simulation? If so, how?
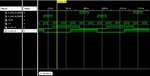