adambose1990
Newbie level 4
- Joined
- Oct 7, 2011
- Messages
- 7
- Helped
- 0
- Reputation
- 0
- Reaction score
- 0
- Trophy points
- 1,281
- Location
- Kolkata, India
- Activity points
- 1,389
Hi guys, here is a simple project I have done in 89s52 IC of 8051 series. Making a Digital Clock.
Components required:
1 microcontroller 89C52(89S52 will also do)
2 ceramic capacitors-22pF
1 switch(button for reset purpose)
1 electrolytic capacitor-10uF,25V
1 crystal oscillator-11.0592MHz
16x2 LCD display
1 resistor-10k
Software you will need:
The programming of the microcontroller is done using keil compiler. Port 2 is output port, This LCD is in 8bit mode.
Details of LCD you can have here.
**broken link removed**
Circuit Diagram:
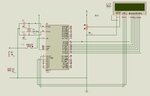
The detail explanation of the code is done below:
CODE:
But before that you have to make lcd.h file. Its codes are given also here. This Header is specially made for this program.
CODE:
Thanks a lot...
For any quarries you can contact adambose1990@gmail.com
You can download the following attachents
.Hex file
.H file
.C file // for keil
.DSN file // for proteus
They are all in rar file. Extract them all.
View attachment Desktop.rar
Components required:
1 microcontroller 89C52(89S52 will also do)
2 ceramic capacitors-22pF
1 switch(button for reset purpose)
1 electrolytic capacitor-10uF,25V
1 crystal oscillator-11.0592MHz
16x2 LCD display
1 resistor-10k
Software you will need:
The programming of the microcontroller is done using keil compiler. Port 2 is output port, This LCD is in 8bit mode.
Details of LCD you can have here.
**broken link removed**
Circuit Diagram:
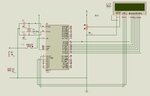
The detail explanation of the code is done below:
CODE:
Code C - [expand] 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 //main.c #include <REG51.H> #include "lcd.h" void ms_delay(int time) { unsigned int i,j; for(i=0;i<time; i++) for(j=0;j<=1275;j++); } void main(void) { int hr=00,min=00,sec=00,flag=0; LCD_init(); LCD_putnum(hr,2); //|HH:MM:SS AM LCD_sendstring(":"); LCD_putnum(min,2); LCD_sendstring(":"); LCD_putnum(sec,2); LCD_sendstring(" AM"); while(1) { for (sec=0;sec<60;sec++) { LCD_gotoxy(6,0); LCD_putnum(sec,2); ms_delay(142); if (sec==59) { min=min+1; if(min==60) { if(hr==11&&sec==59) { hr=0; min=0; sec=-1; flag=~flag; LCD_gotoxy(0,0); LCD_putnum(hr,2); LCD_gotoxy(3,0); LCD_putnum(min,2); LCD_gotoxy(10,0); if (flag==0) LCD_sendstring("AM"); else LCD_sendstring("PM"); } else { hr=hr+1; min=0; LCD_gotoxy(0,0); LCD_putnum(hr,2); LCD_gotoxy(3,0); LCD_putnum(min,2); } } LCD_gotoxy(3,0); LCD_putnum(min,2); } } } }
But before that you have to make lcd.h file. Its codes are given also here. This Header is specially made for this program.
CODE:
Code C - [expand] 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 //lcd.h #ifndef __LCD_H__ #define __LCD_H__ #define LCD_data P2 void _LCD_busy(void); void _LCD_sdelay(void); void _LCD_poweron(void); void _LCD_command(unsigned char); void _LCD_senddata(unsigned char); void _LCD_sendstring(unsigned char *); void _LCD_putnum(int, int); void _LCD_init(void); void _LCD_clear(void); sbit LCD_rs=P1^0; sbit LCD_rw=P1^1; sbit LCD_en=P1^2; void LCD_busy() { unsigned char i,j; for(i=0;i<50;i++) for(j=0;j<255;j++); } void LCD_sdelay() { unsigned char i; for(i=0;i<50;i++); } void LCD_poweron() { unsigned int i; for (i=0;i<22500; i++); } void LCD_command(unsigned char var) { LCD_data = var; //Function set: 2 Line, 8-bit, 5x8 dots LCD_rs = 0; //Selected command register LCD_rw = 0; //We are writing in instruction register LCD_en = 1; //Enable H->L LCD_en = 0; LCD_busy(); //Wait for LCD to process the command } void LCD_putnum(int number,int width)// LCD_putnum: Implementing integer value from -99999 to 99999 { unsigned char digit; if (number < 0) { LCD_senddata('-'); LCD_sdelay(); number = -1 * number; } switch (width) { case 5: digit = '0'; while(number >= 10000) // Keep Looping for larger than 10 { digit++; // Increase ASCII character number -= 10000; // Subtract number with 10 } LCD_senddata(digit); // Put the Second digit LCD_sdelay(); case 4: digit = '0'; while(number >= 1000) // Keep Looping for larger than 10 { digit++; // Increase ASCII character number -= 1000; // Subtract number with 10 } LCD_senddata(digit); // Put the Second digit LCD_sdelay(); case 3: digit = '0'; while(number >= 100) // Keep Looping for larger than 10 { digit++; // Increase ASCII character number -= 100; // Subtract number with 10 } LCD_senddata(digit); // Put the Second digit LCD_sdelay(); case 2: digit = '0'; while(number >= 10) // Keep Looping for larger than 10 { digit++; // Increase ASCII character number -= 10; // Subtract number with 10 } LCD_senddata(digit); // Put the Second digit LCD_sdelay(); case 1: LCD_senddata('0' + number); // Put the First digit LCD_sdelay(); } } void LCD_sendstring(unsigned char *var) { while(*var) //till string ends LCD_senddata(*var++); //send characters one by one } void LCD_senddata(unsigned char var) { P2 = var; //Function set: 2 Line, 8-bit, 5x7 dots LCD_rs = 1; //Selected data register LCD_rw = 0; //We are writing LCD_en = 1; //Enable H->L LCD_en = 0; //LCD_busy(); //Wait for LCD to process the command } void LCD_init(void) { LCD_data = 0x38; //Function set: 2 Line, 8-bit, 5x8 dots LCD_rs = 0; //Selected command register LCD_rw = 0; //We are writing in data register LCD_en = 1; //Enable H->L LCD_en = 0; LCD_busy(); //Wait for LCD to process the command LCD_data = 0x0C; //Display on, Curson blinking off command LCD_rs = 0; //Selected command register LCD_rw = 0; //We are writing in data register LCD_en = 1; //Enable H->L LCD_en = 0; LCD_busy(); //Wait for LCD to process the command LCD_data = 0x01; //Clear LCD LCD_rs = 0; //Selected command register LCD_rw = 0; //We are writing in data register LCD_en = 1; //Enable H->L LCD_en = 0; LCD_busy(); //Wait for LCD to process the command LCD_data = 0x06; //Entry mode, auto increment with no shift LCD_rs = 0; //Selected command register LCD_rw = 0; //We are writing in data register LCD_en = 1; //Enable H->L LCD_en = 0; //Enable H->L LCD_busy(); } void LCD_clear(void) { LCD_data = 0x01; //Clear LCD LCD_rs = 0; //Selected command register LCD_rw = 0; //We are writing in data register LCD_en = 1; //Enable H->L LCD_en = 0; LCD_busy(); } void LCD_gotoxy(int x, int y)//x=0..16, y= 0..1 { int dr; if (y==0) dr=x+0x80; if (y==1) dr=x+0xC0; LCD_data = dr; //Function set: 2 Line, 8-bit, 5x8 dots LCD_rs = 0; //Selected command register LCD_rw = 0; //We are writing in instruction register LCD_en = 1; //Enable H->L LCD_en = 0; LCD_busy(); } #endif
Thanks a lot...
For any quarries you can contact adambose1990@gmail.com
You can download the following attachents
.Hex file
.H file
.C file // for keil
.DSN file // for proteus
They are all in rar file. Extract them all.
View attachment Desktop.rar