ktsangop
Junior Member level 1
verilog generate
Hi everybody!
I have the following code that works as a shift register :
module sreg (C, SI, SO);
input C,SI;
output SO;
reg [7:0] tmp;
always @(posedge C)
begin
tmp = tmp << 1;
tmp[0] = SI;
end
assign SO = tmp[7];
endmodule
And I want to create multiple instances of the sreg module which are interconnected with eachother. They will all have the same clock and do the same job plus the output of every shift register will provide the input to the next one. Just like the image below :
Can anybody please help me on how to do this using the generate/endgenerate statements in verilog?
I understand that i have to put them all inside a higher level module, but cannot find out how the ports will be connected.
Thanks in advance!
Hi everybody!
I have the following code that works as a shift register :
module sreg (C, SI, SO);
input C,SI;
output SO;
reg [7:0] tmp;
always @(posedge C)
begin
tmp = tmp << 1;
tmp[0] = SI;
end
assign SO = tmp[7];
endmodule
And I want to create multiple instances of the sreg module which are interconnected with eachother. They will all have the same clock and do the same job plus the output of every shift register will provide the input to the next one. Just like the image below :
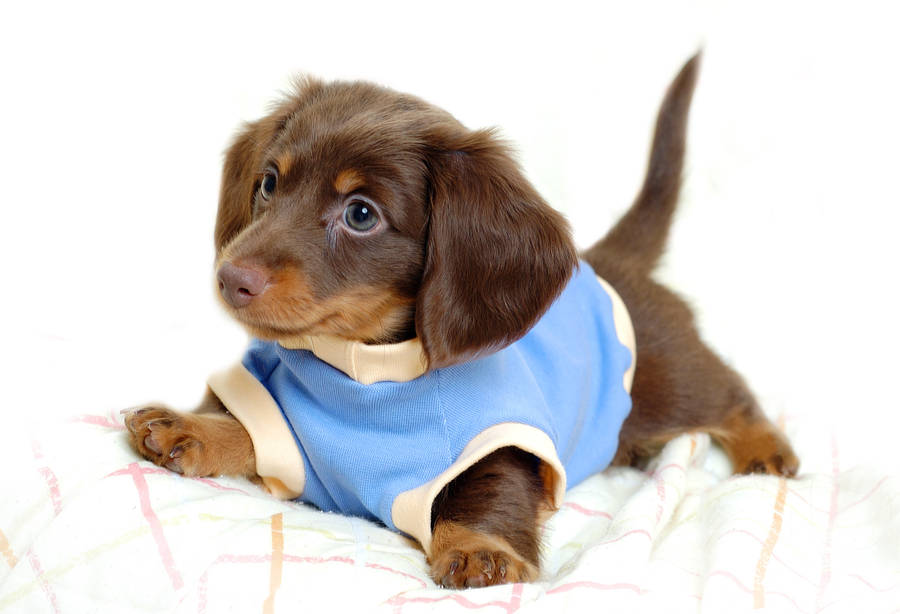
Can anybody please help me on how to do this using the generate/endgenerate statements in verilog?
I understand that i have to put them all inside a higher level module, but cannot find out how the ports will be connected.
Thanks in advance!