lloydi12345
Member level 4
I know there are many threads on how to build these projects but I really would like to know the basics. It feels better to make my own code without copying and pasting from others work. I already understand how to use shift register 595 and I'm scanning the LEDs with a johnson counter 4022. I'm already done with displaying static letter.
So far here it is:
Although the letter is not perfect it's already good for me.
Here's my code:
Now I would like to scroll it from right to left. Can someone give me an idea how to do it? Basically my problem is the algorithm involved. I know I will be using logical operations like OR and then do some shifting but don't know how to start. Thanks.
Cheers, :-D
lloydi
So far here it is:
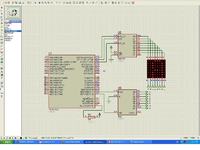
Although the letter is not perfect it's already good for me.
Here's my code:
Code:
/*
Trial1 displaying matrix
*/
sbit clock_a at rc0_bit;
sbit data_a at rc1_bit;
sbit latch_a at rc2_bit;
sbit clock_b at rd6_bit;
sbit reset at rd7_bit;
sbit enable at rd5_bit;
const unsigned short arrays[][8] = {{0xFF,0xFF,0x80,0x77,0x77,0x77,0x80,0xFF}
};
unsigned int scanner, scanner2, scantime, disp, row, column, mask, repeat;
void main() {
LATC = 0x00;
CMCON = 0x07;
ADCON0 = 0x00;
ADCON1 = 0x0F;
TRISC = 0x00;
TRISD = 0x00;
scanner2 = 0;
while(1) {
for(column=0;column<1;column++){
for (repeat=0;repeat<80;repeat++){
for(row=0;row<8;row++){
disp = arrays[column][row];
mask = 0x01;
for (scantime=0;scantime<8;scantime++){
scanner = mask & disp;
if (scanner==0)
data_a = 0;
else data_a = 1;
mask = mask << 1;
clock_a = 1;
clock_a = 0;
}
latch_a = 1;
latch_a = 0;
clock_b = 1;
clock_b = 0;
delay_us(100);
}
}
}
}
}
Now I would like to scroll it from right to left. Can someone give me an idea how to do it? Basically my problem is the algorithm involved. I know I will be using logical operations like OR and then do some shifting but don't know how to start. Thanks.
Cheers, :-D
lloydi
Last edited: